How to query data with GraphQL in Oracle Content Management
June 9, 2021In recent years, GraphQL has become a popular means of addressing some of the performance drawbacks inherent to REST APIs and the heavier response payloads associated with traditional approaches to headless content delivery. GraphQL is a query language that addresses response bloat by allowing queries to specify their data requirements and tailor them to their needs rather than accepting what the server provides willy-nilly.
Servers exposing a GraphQL API also emit data that matches the GraphQL query’s structure, granting the client unprecedented control over the chattiness of their communication with an upstream data source. The GraphQL ecosystem provides GraphiQL, a browser-based development environment for debugging and testing queries as well as introspecting the entire schema outlined by a GraphQL API. GraphQL is today a fixture of many JavaScript and Jamstack ecosystems, and Oracle Content Management now offers full support for GraphQL read queries.
At Oracle Content Management, we recently introduced a new GraphQL API for content delivery that includes support for all content published through a channel and all asset types. In this article, I’ll walk through some of the most salient and impressive features that comprise our GraphQL API and some examples of the unique power developers working with Oracle Content Management as a headless CMS in technologies like React can now access. I’ve also created a short video if you’re pressed on time and a longer, in-depth video with a more complete exploration.
Introspecting the GraphQL schema
In order to begin using GraphQL in Oracle Content Management, you need to have an existing content model with several assets (i.e. content items) that are published to a channel. For more information about these steps, I have a quickstart guide available for developers. You can also set up a React application to use in conjunction with Oracle Content Management, but this isn’t required for this tour.
To access the GraphiQL interface and the documentation explorer, navigate to the following URL on your own instance:
https://{instance_url}/content/published/api/v1.1/graphql/explorer
If you open the documentation explorer in the upper right, you’ll see a fully introspectable schema that reflects the current state of your content model. Like most other headless CMSs that provide a GraphQL API for developers, this schema evolves automatically to reflect the changes you make to your content schema. In the screenshot below, we see a content type,
pressRelease
, with several custom fields (pressReleaseFields
) defined therein, such as author
(a reference field) and masthead
(a digital asset field).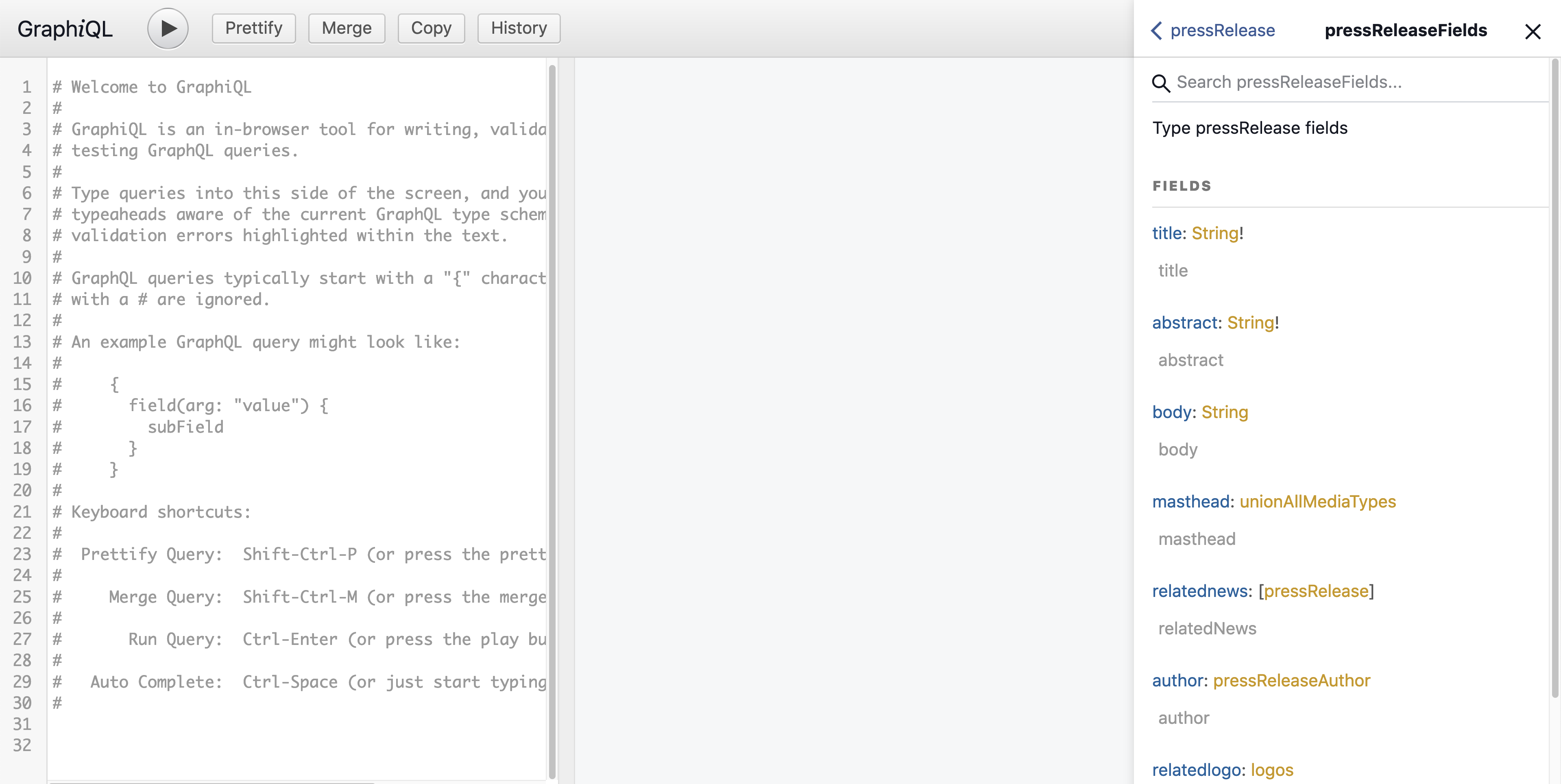
Querying an individual asset
GraphQL queries can be anonymous or named, but the most common approach for a typical GraphQL query is to keep them anonymous. The following query targets a top-level field (
getPressRelease
), which fetches an individual press release content item. Required arguments for all of these top-level fields are channelToken
(an authentication token for an Oracle Content Management channel) and one of either id
or slug
, both of which are found in every content item. The channelToken
value can also be provided outside the query as a header in the HTTP request surrounding the GraphQL query.The following query uses an
id
and channelToken
to acquire certain core fields. To execute a query in GraphiQL, either click “Run” in the toolbar or type Command+Enter.{
getPressRelease(
id: "CORE1FADA80EEACE4B4A84B76C07A931B317",
channelToken: "573ae0bcb95347d283cdbea8a4d29641"
) {
id
slug
name
description
}
}
Congratulations! You’ve issued your first GraphQL query against Oracle Content Management. To use the slug to target an individual item, instead, change the
id
argument to a slug
argument:{
getPressRelease(
slug: "bed-bath-beyond-selects-oracle-to-modernize-enterprise",
channelToken: "573ae0bcb95347d283cdbea8a4d29641"
) {
id
slug
name
description
}
}
The results of this query can be seen in the screenshot below.
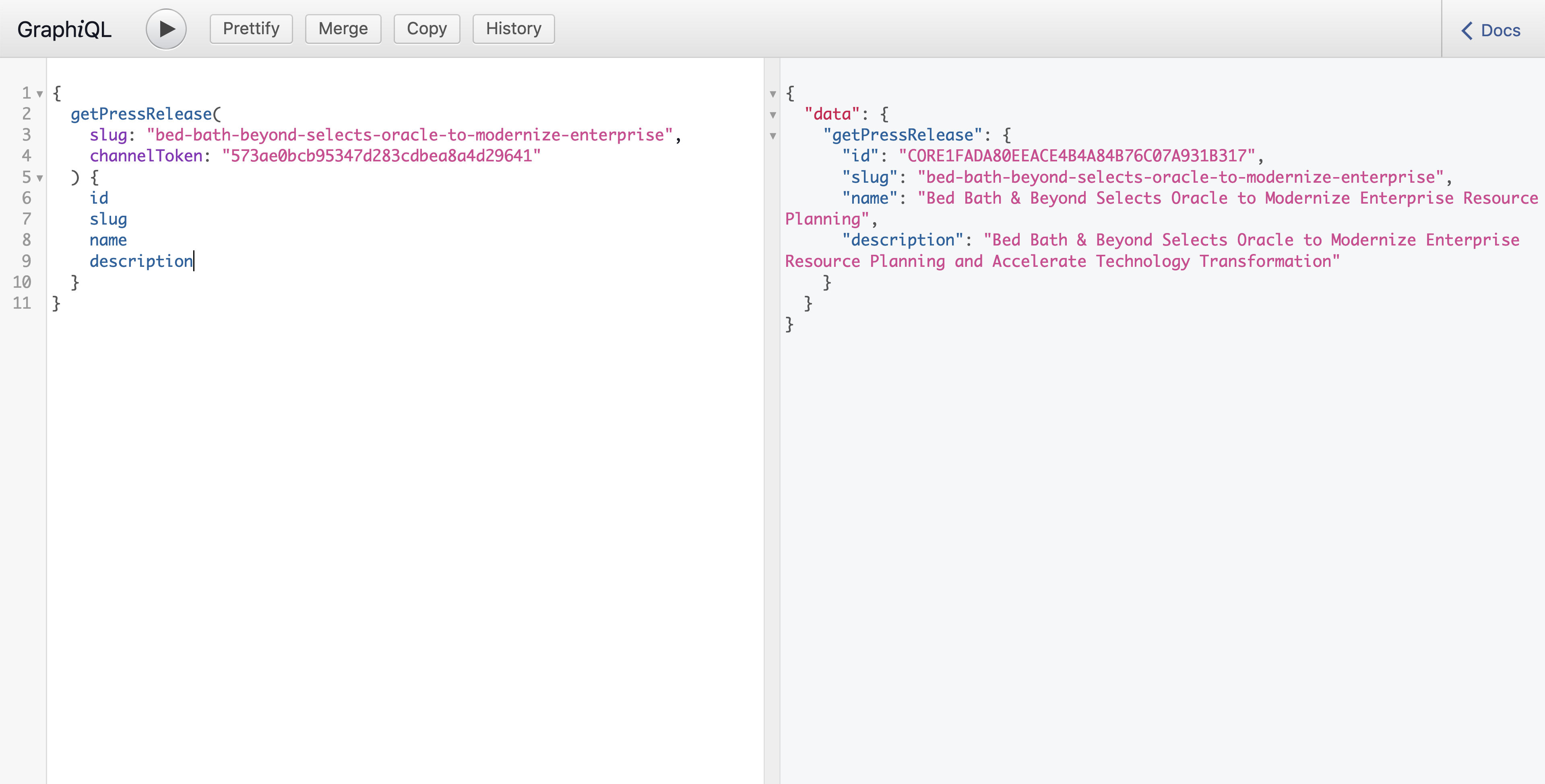
Within the individual asset, you can retrieve custom fields in the content type that are defined in the content model in a query such as the following:
{
getPressRelease(
slug: "bed-bath-beyond-selects-oracle-to-modernize-enterprise",
channelToken: "573ae0bcb95347d283cdbea8a4d29641"
) {
id
slug
name
description
fields {
title
abstract
body
}
}
}
The screenshot below illustrates the result of this query containing fields on the content type.
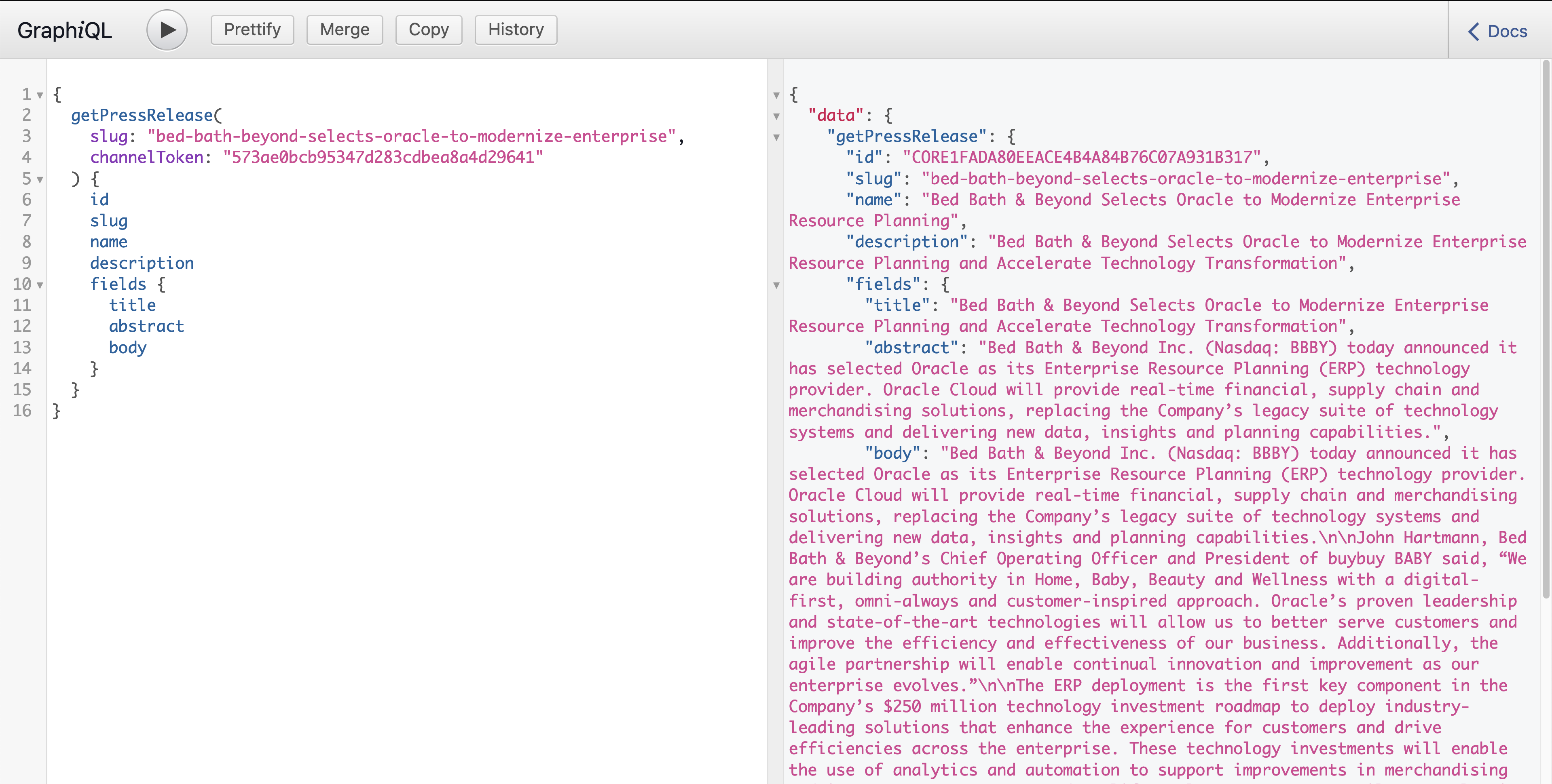
Querying a referenced asset
The GraphQL API for content delivery in Oracle Content Management also permits you to access an asset’s referenced data through a referenced asset. For instance, the query below retrieves the referenced author content type together with the user-defined fields in the press release content type. This nested structure reflects how these referenced assets can be accessed through the Oracle Content Management REST APIs as well.
{
getPressRelease(
slug: "bed-bath-beyond-selects-oracle-to-modernize-enterprise",
channelToken: "573ae0bcb95347d283cdbea8a4d29641"
) {
id
slug
name
description
fields {
title
abstract
body
author {
fields {
firstname
lastname
bio
}
}
}
}
}
The screenshot below illustrates the result of this query containing referenced fields.
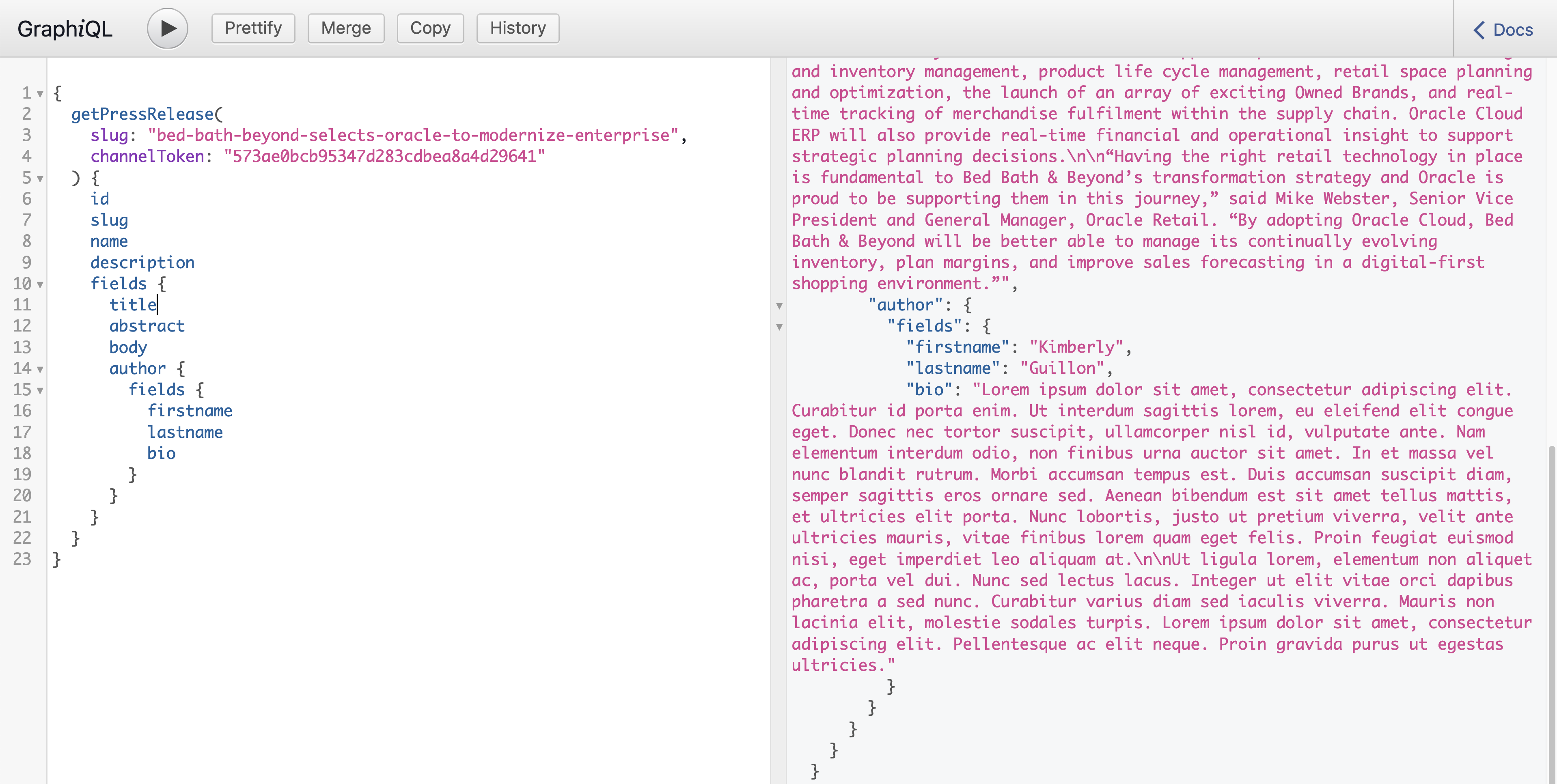
Note that the number of nested levels for referenced assets permitted within a top-level query is ten; this means that at most ten levels of child references can be retrieved using a single query.
Querying renditions of digital assets
Finally, one of Oracle Content Management’s most powerful features is its support for image renditions, which allows developers to retrieve URLs representing arbitrary versions of certain media assets for the purposes of their application. For images, this means you can retrieve thumbnail, medium-sized, and hero versions of your image assets depending on how you have defined them within your Oracle Content Management instance.
This query retrieves the URL for a “Large” rendition of an individual image asset in addition to the other fields previously fetched:
{
getPressRelease(
slug: "bed-bath-beyond-selects-oracle-to-modernize-enterprise",
channelToken: "573ae0bcb95347d283cdbea8a4d29641"
) {
id
slug
name
description
fields {
title
abstract
body
author {
fields {
firstname
lastname
bio
}
}
masthead {
...headerImage
}
}
}
}
fragment headerImage on image {
id
fields {
rendition(name: "Large", format: "jpg") {
file {
url
}
}
}
}
You can also list various renditions (in both
jpg
and webp
formats) using the renditions field:fragment headerImages on image {
id
fields {
renditions {
file {
url
}
}
}
}
The screenshot below illustrates the result of this query containing a fragment that identifies an image rendition.
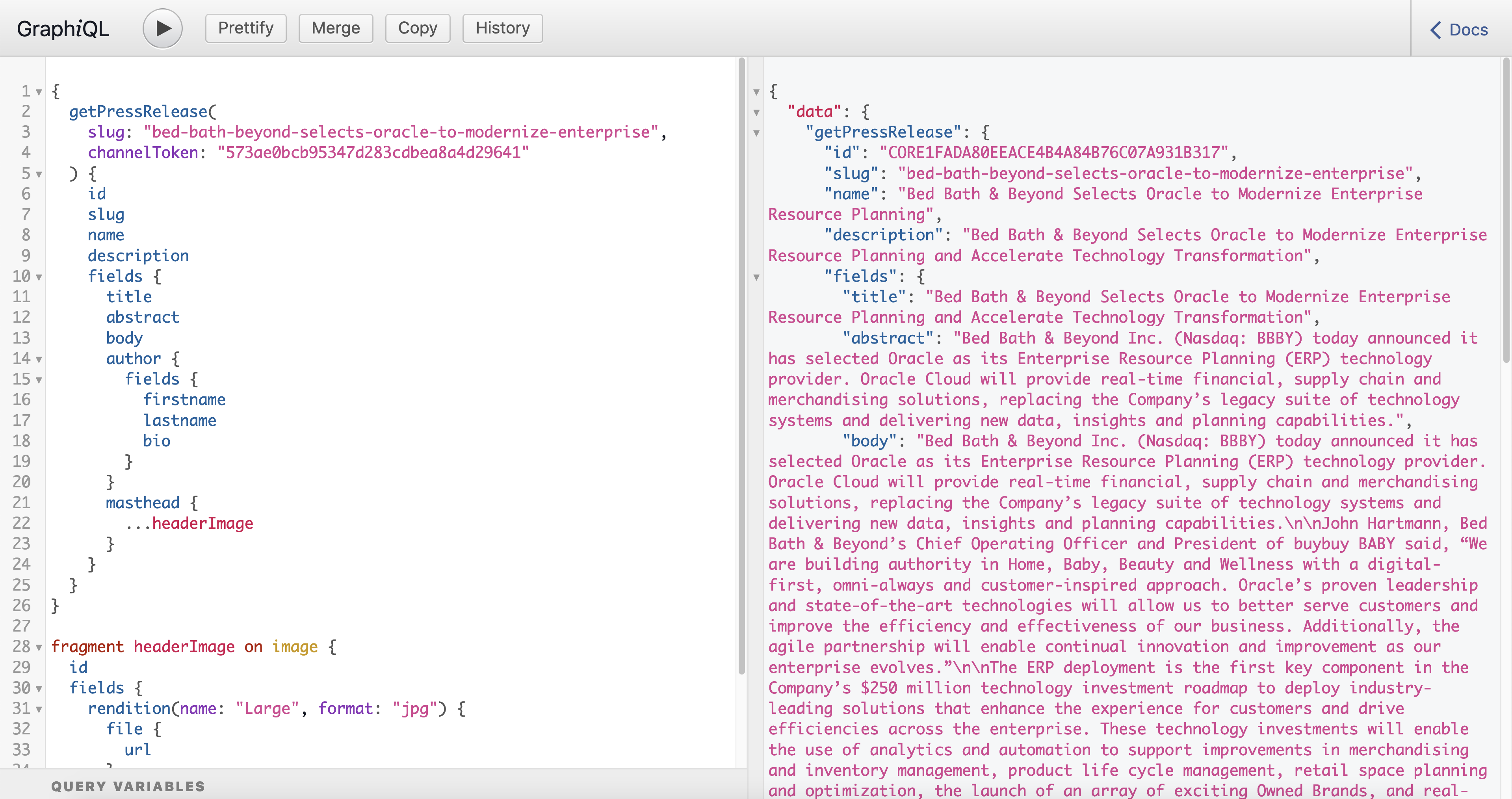
Understanding the schema
Every asset type is represented by a GraphQL type that carries the same name. The documentation explorer provides a comprehensive accounting of all types and all fields available in the GraphQL API for Oracle Content Management. For instance, the type
pressRelease
is an implementation of the item
interface:interface item {
createdDate: DateTime!
description: String
id: ID!
language: String
name: String!
slug: String
taxonomies: [taxonomy]
translatable: Boolean!
type: String!
typeCategory: String
updatedDate: DateTime!
variation(variationName: String, variationType: String!): item
variations(variationType: String!): [item]
}
The
pressRelease
type implements the item
type and also references the pressReleaseFields
type representing custom-defined pressRelease
fields on the content type, mirroring the expression of these fields in the REST APIs in Oracle Content Management:type pressRelease implements item {
id: ID!
name: String!
description: String
createdDate: DateTime!
updatedDate: DateTime!
type: String!
typeCategory: String
language: String
slug: String
translatable: Boolean!
fields: pressReleaseFields!
taxonomies: [taxonomy]
variation(variationName: String, variationType: String!): pressRelease
variations(variationType: String!): [pressRelease]
}
type PressReleaseFields {
abstract: String!
author: pressReleaseAuthor
body: String
masthead: unionAllMediaTypes
relatedLogo: logos
relatedNews: [pressRelease]
settings: JSON
title: String!
}
The names of the GraphQL types represent the API name of respective types in Oracle Content Management. These names are automatically assigned, as close the name as possible, prefixing where needed so as to avoid conflicts and collisions with reserved words in GraphQL.
Conclusion
As of this writing, the GraphQL API for content delivery in Oracle Content Management is available in all current instances of our CMS, with full support for all of the queries we covered in this overview. For developers working with consumer technologies such as Gatsby and React, this now means that you can take advantage of GraphQL or our REST APIs as you prefer to drive the data requirements of your application. For instance, Gatsby developers can now depend on either our
plugin for sourcing data or use gatsby-source-oce
gatsby-source-graphql
against the GraphQL API on Oracle Content Management.This is just the beginning of some of the exciting features we have coming in the roadmap for Oracle Content Management’s support for GraphQL. Soon, we’ll share even more information about how to access other features in GraphQL and manipulate the returned data in ways that go well beyond this brief summary. If you’re using GraphQL to drive content delivery to your application, we’d love to hear from you and learn more about how you’d like to see our GraphQL API better suit your needs.
For even more information about leveraging Oracle Content Management as a headless CMS, consult our documentation and check out my guides to getting started with Oracle Content Management and building a React application consuming Oracle Content Management.